ChatGPT4とMidJourneyで「今日の料理とレシピ」を提案してくれるWEBアプリを制作
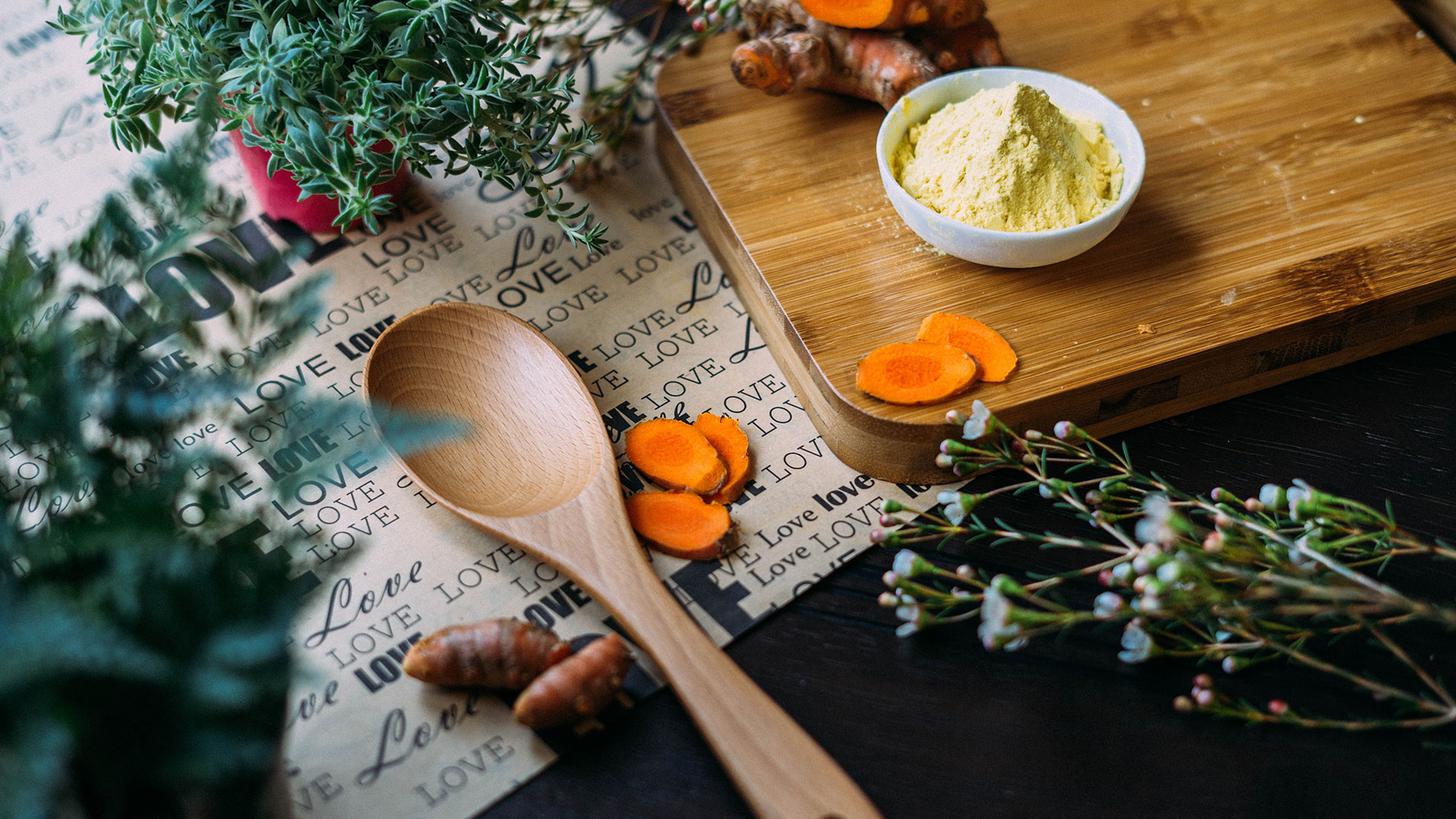
先日「ChatGPT4を使ってシンプルなブロック崩しゲームを作成」という記事で、ChatGPT4と一緒にプログラムしてみましたが、今日はブロック崩しゲームに続き、簡単なWEBアプリを制作してみたいと思います。
今回は、今日、何を食べたいか、結構頻繁に考えることのような気がするので、「今日の御飯とレシピ」を提案してくれるWEBアプリをChatGPT4と制作していきたいと思います。
まずは人格と開発要件を提示
ブロック崩しと同じくまずはプロンプトを書きます。
あなたは「優秀なプログラマー」です。以下の前提条件をもとに、「今日の料理とレシピ」を提案してくれるWEBアプリのコードを書いてください。
前提条件
・HTML+CSS+JSで完結する
・コードの行数を少なくシンプルに
・料理データは10件
・表示されるデータは画像、料理名、レシピ
・オープンソースは利用可能
・APIは利用しない
最高の結果を得るために、情報が不足するようであれば質問してください。
初期のコード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>今日の料理とレシピ</title>
<style>
body {
font-family: sans-serif;
}
img {
max-width: 100%;
}
</style>
</head>
<body>
<h1>今日の料理とレシピ</h1>
<div id="recipe-container">
<img id="recipe-image" src="" alt="料理の画像">
<h2 id="recipe-name">料理名</h2>
<ol id="recipe-steps"></ol>
</div>
<button onclick="displayRecipe()">別の料理を表示</button>
<script>
const recipes = [
{
name: '料理1',
img: 'img1.jpg',
steps: ['手順1', '手順2', '手順3']
},
// 他の9件の料理データも同様に追加
];
function displayRecipe() {
const recipe = recipes[Math.floor(Math.random() * recipes.length)];
document.getElementById('recipe-image').src = recipe.img;
document.getElementById('recipe-name').textContent = recipe.name;
const steps = document.getElementById('recipe-steps');
steps.innerHTML = '';
for (const step of recipe.steps) {
const li = document.createElement('li');
li.textContent = step;
steps.appendChild(li);
}
}
displayRecipe();
</script>
</body>
</html>
HTMLにすべてが書かれて出力されました。データもダミーデータとなっているため、HTMLとCSS、javascriptは分けて、さらに具体的なデータが入るようにChatGPTに依頼
ファイル分割と実データの作成
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>今日の料理とレシピ</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>今日の料理とレシピ</h1>
<div id="recipe-container">
<img id="recipe-image" src="" alt="料理の画像">
<h2 id="recipe-name">料理名</h2>
<ol id="recipe-steps"></ol>
<button onclick="displayRecipe()">別の料理を表示</button>
</div>
<script src="scripts.js"></script>
</body>
</html>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: sans-serif;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
flex-direction: column;
}
h1 {
margin-bottom: 1rem;
font-size: 2rem;
text-align: center;
color: #333;
}
img {
max-width: 100%;
display: block;
margin-bottom: 1rem;
}
#recipe-container {
background-color: #fff;
padding: 1.5rem;
border-radius: 5px;
box-shadow: 0 2px 6px rgba(0, 0, 0, 0.1);
max-width: 500px;
width: 100%;
}
#recipe-name {
font-size: 1.5rem;
color: #333;
margin-bottom: 1rem;
text-align: center;
}
#recipe-steps {
color: #666;
margin-bottom: 1rem;
}
button {
background-color: #008CBA;
color: #fff;
font-size: 1rem;
padding: 0.5rem 1rem;
border-radius: 3px;
border: none;
cursor: pointer;
}
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: sans-serif;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
flex-direction: column;
}
h1 {
margin-bottom: 1rem;
font-size: 2rem;
text-align: center;
color: #333;
}
img {
max-width: 100%;
display: block;
margin-bottom: 1rem;
}
#recipe-container {
background-color: #fff;
padding: 1.5rem;
border-radius: 5px;
box-shadow: 0 2px 6px rgba(0, 0, 0, 0.1);
max-width: 500px;
width: 100%;
}
#recipe-name {
font-size: 1.5rem;
color: #333;
margin-bottom: 1rem;
text-align: center;
}
#recipe-steps {
color: #666;
margin-bottom: 1rem;
}
button {
background-color: #008CBA;
color: #fff;
font-size: 1rem;
padding: 0.5rem 1rem;
border-radius: 3px;
border: none;
cursor: pointer;
}
const recipes = [
{
name: 'オムライス',
img: 'omurice.png,
steps: [
'玉ねぎをみじん切りにする',
'フライパンで玉ねぎを炒める',
'ご飯とケチャップを加えて炒める',
'別のフライパンで卵を焼く',
'卵をご飯の上にのせる'
]
},
{
name: 'カルボナーラ',
img: 'carbonara.png,
steps: [
'スパゲッティを茹でる',
'ベーコンをフライパンで炒める',
'卵、粉チーズ、黒コショウを混ぜる
'炒めたベーコンに茹でたスパゲッティを加える',
'卵の混ぜ物を加え、よく混ぜる'
]
},
{
name: '寿司',
img: 'sushi.png,
steps: [
'酢飯を作る',
'刺身を薄くスライスする',
'手に酢水をつけ、酢飯を握る',
'刺身を酢飯の上にのせる',
'わさびと醤油で食べる'
]
}
];
function displayRecipe() {
const recipe = recipes[Math.floor(Math.random() * recipes.length)];
document.getElementById('recipe-image').src = recipe.img;
document.getElementById('recipe-name').textContent = recipe.name;
const steps = document.getElementById('recipe-steps');
steps.innerHTML = '';
for (const step of recipe.steps) {
const li = document.createElement('li');
li.textContent = step;
steps.appendChild(li);
}
}
displayRecipe();
画像を作成
画像が足りないのでMidJourneyを利用して、データに入っているオムライス、カルボナーラ、寿司の画像を作成。
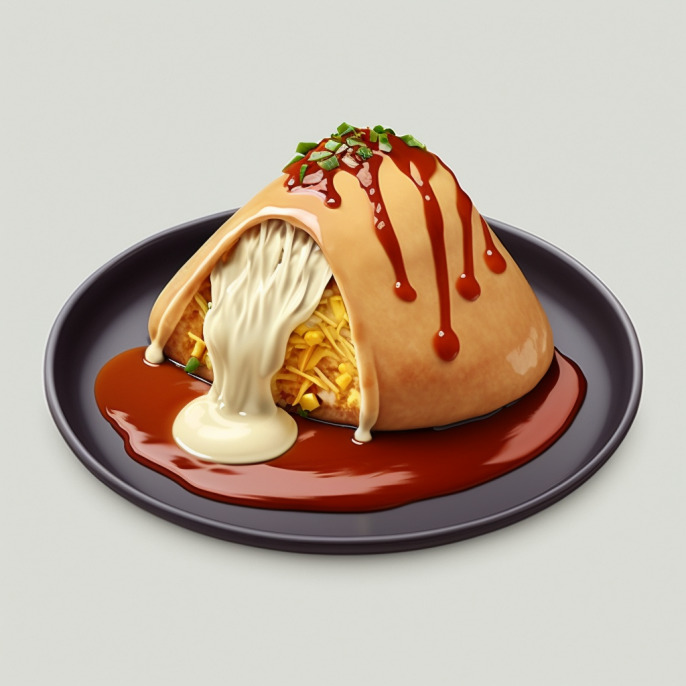
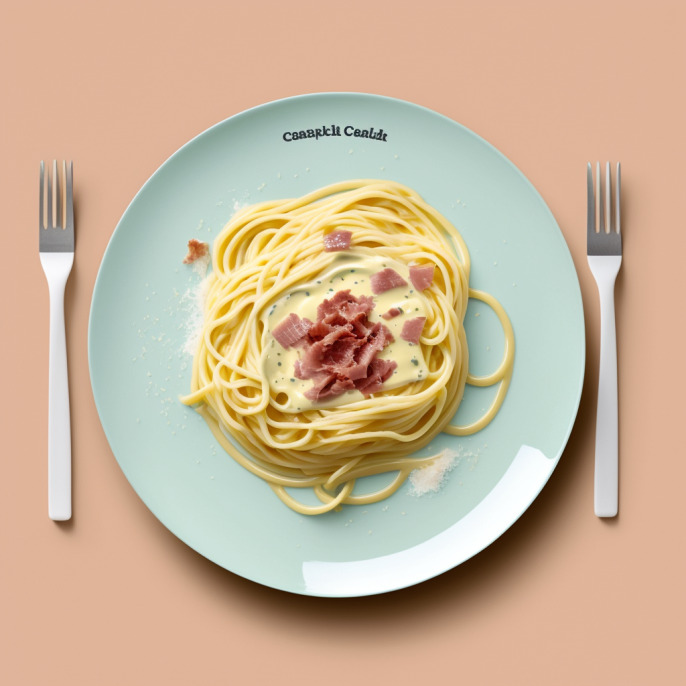

完成したWEBアプリ
実際サーバー上に設置したアプリは以下となります。
今日の料理とレシピ
https://taziku.co.jp/app/menu_v1/
今後の改善ポイント
このWEBアプリについてはある程度のレベルまで引き上げることができるかを、引き続きチャレンジしたいと思います。次回は以下の点を改善しながらレポートいたします。
・レシピなどのデータはJSONで管理 – 将来的なAPI化にも対応させる
・デザインをデザイナーがデザインし整える
・料理の画像をもっと美味しそうに出力
・モバイルに対応
・レシピデータをChatGPTで制作し実用に耐えれるデータ量とする
ChatGPTでプログラムのハードルは間違いなく下がりますね。簡単なものなら人間が書くより早いと思います。もちろん及ばない部分は多々ありますが、プログラムを諦めた人が、創りたいものを創れる世界になるのはとても良いことだと思います。
引き続き可能性を探りながら様々なものを作成していこうと思います。