Claude 3 で書くクリエイティブコーディング
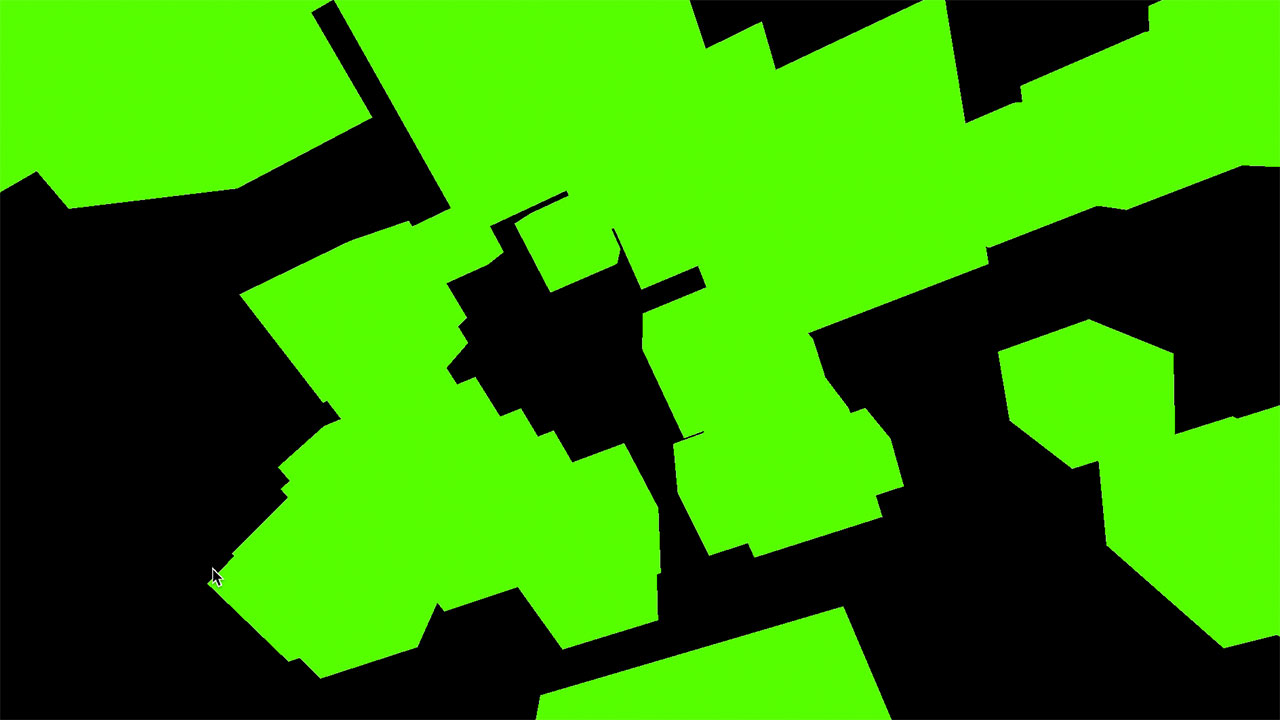
非常に精度が高い、Claude 3、その精度は日本語だけで無く、プログラミングの側面でもかなりの精度を誇っています。今回はClaude 3を活用することで、より直感的かつクリエイティブなアプローチのコーディングを自然言語で行うことが可能です。
今回はThree.jsを指定した上でコーディングに挑戦してみました。
生成したプログラム
今回は立方体のキューブが空間を飛び交いつつ、マウスの位置によって立方体の色を変えるというアニメーションを自然言語でClaude 3に依頼。Claude 3は、シンプルな自然言語で内容を理解し、対応するコードを自動的に生成します。
実際のコード
<!DOCTYPE html>
<html>
<head>
<title>Creative Animation with Three.js</title>
<style>
body { margin: 0; }
canvas { width: 100%; height: 100%; }
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
<script>
// シーンの作成
const scene = new THREE.Scene();
// カメラの設定
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
// レンダラーの設定
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 立方体の作成
const geometry = new THREE.BoxGeometry(1, 1, 1);
const cubes = [];
for (let i = 0; i < 50; i++) {
const materials = [
new THREE.MeshBasicMaterial({ color: 0xff0000, transparent: true, opacity: 0.8 }),
new THREE.MeshBasicMaterial({ color: 0x00ff00, transparent: true, opacity: 0.6 }),
new THREE.MeshBasicMaterial({ color: 0x0000ff, transparent: true, opacity: 0.4 }),
new THREE.MeshBasicMaterial({ color: 0xffff00, transparent: true, opacity: 0.6 }),
new THREE.MeshBasicMaterial({ color: 0xff00ff, transparent: true, opacity: 0.8 }),
new THREE.MeshBasicMaterial({ color: 0x00ffff, transparent: true, opacity: 1.0 })
];
const cube = new THREE.Mesh(geometry, materials);
cube.position.x = Math.random() * 10 - 5;
cube.position.y = Math.random() * 10 - 5;
cube.position.z = Math.random() * 10 - 5;
scene.add(cube);
cubes.push(cube);
}
// 境界線の作成
const edgesMaterial = new THREE.LineBasicMaterial({ color: 0xffffff });
cubes.forEach((cube) => {
const edges = new THREE.EdgesGeometry(cube.geometry);
const line = new THREE.LineSegments(edges, edgesMaterial);
cube.add(line);
});
// アニメーションループ
function animate() {
requestAnimationFrame(animate);
cubes.forEach((cube, index) => {
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
cube.position.y = Math.sin(Date.now() * 0.001 + index * 0.1) * 2;
});
renderer.render(scene, camera);
}
// マウスムーブイベントの処理
document.addEventListener('mousemove', onDocumentMouseMove, false);
function onDocumentMouseMove(event) {
const mouseX = (event.clientX / window.innerWidth) * 2 - 1;
const mouseY = -(event.clientY / window.innerHeight) * 2 + 1;
cubes.forEach((cube) => {
const distance = Math.sqrt(Math.pow(mouseX - cube.position.x, 2) + Math.pow(mouseY - cube.position.y, 2));
const hue = Math.floor(distance * 100) % 360;
cube.material.forEach((material) => {
material.color.setHSL(hue / 360, 1, 0.5);
});
});
}
// ウィンドウリサイズ時の処理
window.addEventListener('resize', onWindowResize, false);
function onWindowResize() {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
}
// アニメーションの開始
animate();
</script>
</body>
</html>
動作デモ
実際の動作については以下をご覧ください。
以下のURLは上記からの修正版-境界線を引き、各面を透明度を変更するように修正
https://taziku.co.jp/c-coding/c-anime01.html
クリエイターもコーディングが可能に
クリエイターがビジョンを言葉で表現すれば、Claude 3がそれを視覚的に表現するためのコードを生成してくれます。プログラミングスキルを持たないクリエイターでも、インタラクティブなデジタルアートを創作することが可能になる世界がすぐそこまで来ています。